Mastering Swift Generics: Simplify Your Code with Reusability
Written on
Chapter 1: Understanding Swift Generics
Generics in Swift can initially appear challenging, but you might have already utilized them without realizing it. They allow for the creation of functions and types that are flexible and reusable across various data types, which can help prevent code duplication.
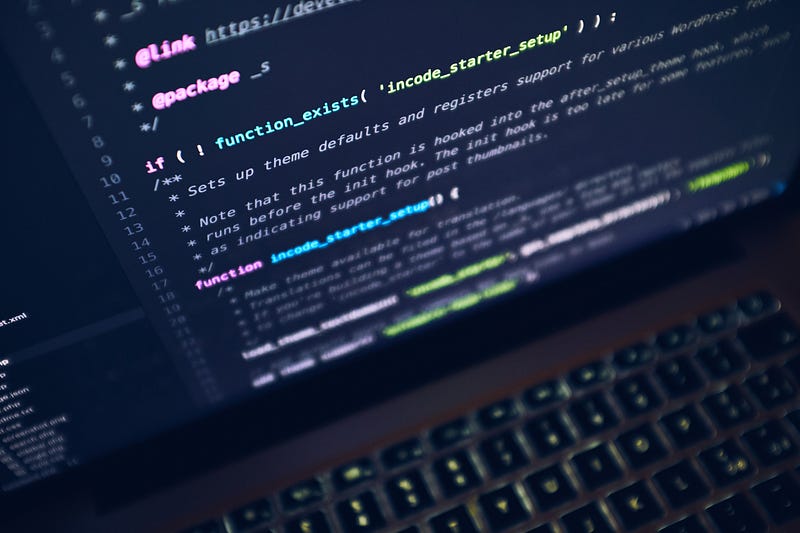
Generics enable you to construct reusable functions and types that can operate with any given type, based on the constraints you establish. This not only makes your code less repetitive but also enhances clarity and abstraction.
Section 1.1: The Purpose of Generics
Consider a scenario where you need to implement a function to print information about an argument of any type, such as a String or an Int. Without generics, you'd have to define multiple functions for each possible data type:
func infoString(_ a: String) {
print("Argument type is String with a value of (a)")
}
func infoInt(_ a: Int) {
print("Argument type is Int with a value of (a)")
}
func infoDouble(_ a: Double) {
print("Argument type is Double with a value of (a)")
}
// ... and so on
This approach leads to excessive repetition and is impractical given the myriad of data types available.
Instead, generics allow you to create a single function that can accept any type:
func info<T>(_ a: T) {
print("Argument type is (type(of: a)) with a value of (a)")
}
Now, you can use this function for various types of inputs:
info(3) // Argument type is Int with a value of 3
info(3.2) // Argument type is Double with a value of 3.2
info("Yeah") // Argument type is String with a value of Yeah
This implementation utilizes a generic placeholder type T, indicating that the function can accept any type. Including <T> after the function name signifies the use of this placeholder.
Section 1.2: Another Use Case for Generics
Imagine you have arrays containing different types, such as integers or strings, and you want to print their contents. With separate functions for each type, the code becomes redundant:
let numbers = [1, 2, 3, 4, 5]
let names = ["Nicolas", "Anne", "Robert", "Sofie"]
func printInts(_ arr: [Int]) {
arr.forEach { print($0) }
}
func printStrings(_ arr: [String]) {
arr.forEach { print($0) }
}
printInts(numbers) // prints all the numbers
printStrings(names) // prints all the names
Instead of duplicating functions, a generic printing function can be utilized:
func arrPrint<T>(_ arr: [T]) {
arr.forEach { print($0) }
}
You can now easily print both arrays:
arrPrint(numbers) // prints all the numbers
arrPrint(names) // prints all the names
Conclusion
By leveraging generics, you can eliminate redundancy and write functions that work seamlessly with any type. Thank you for reading, and happy coding!
Explore the fundamentals of Swift generics in this video, "Swift Generics Made Super Easy for You."
Learn the basics of generics in Swift with the video "Introduction to Generics in Swift."
You may also find useful resources like:
- iOS Development Tips in 2021 | Code Like a Pro | Codingem
- 50 Swift Interview Questions and Answers for 2021
- Swift — Upgrade Your Skills with These 8 Tips
References
For more insights, check out Swift's official documentation: About Swift - The Swift Programming Language (Swift 5.4).