Mastering JavaScript Basics: Interview Prep Day 5 Insights
Written on
Chapter 1: Introduction to Day 5
Welcome to Day 5 of our 100-day JavaScript Interview Preparation journey! Today, we focus on the most frequently asked string manipulation questions in coding interviews. This exploration is aimed at beginners who are eager to enhance their coding skills.
To accompany your learning, check out this online JavaScript Playground for hands-on practice:
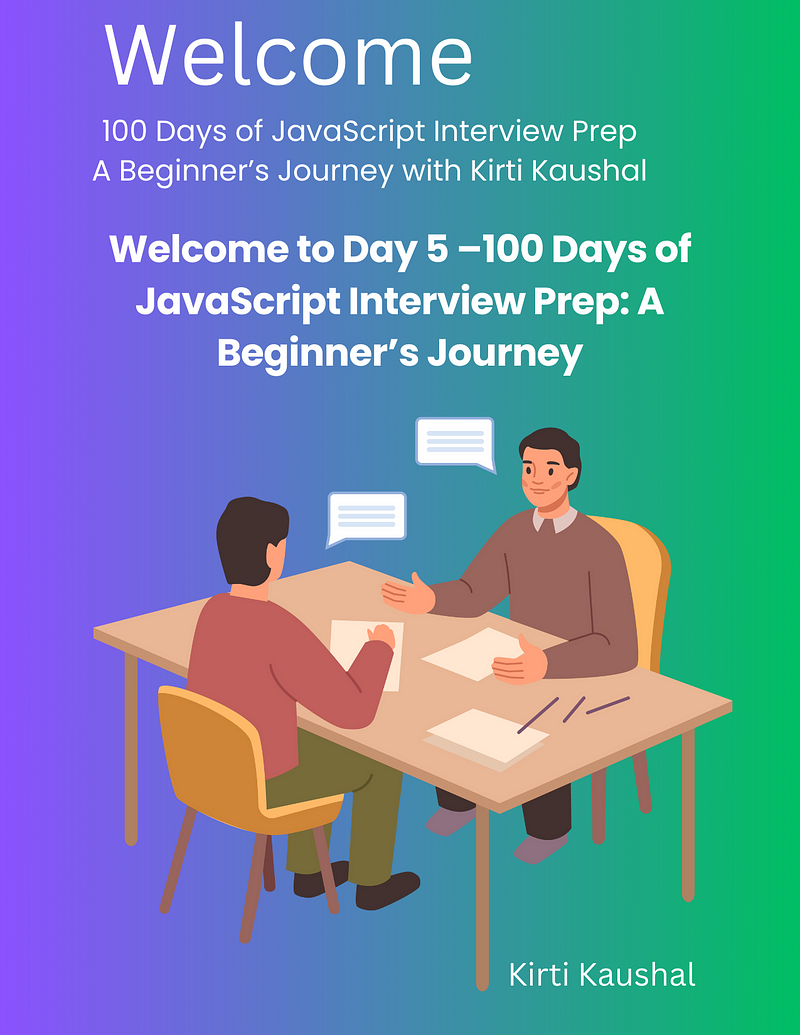
The following sections will address common string manipulation tasks that you might encounter during interviews.
Section 1.1: Capitalizing Strings
Question 1: How do you capitalize a string?
This question may seem straightforward, but it's important to understand the built-in methods we can utilize effectively.
Answer:
You can use the toUpperCase() method in JavaScript to convert all lowercase characters in a string to uppercase. Here's a simple example:
let str = "test";
let newStr = str.toUpperCase();
console.log(newStr); // Output: TEST
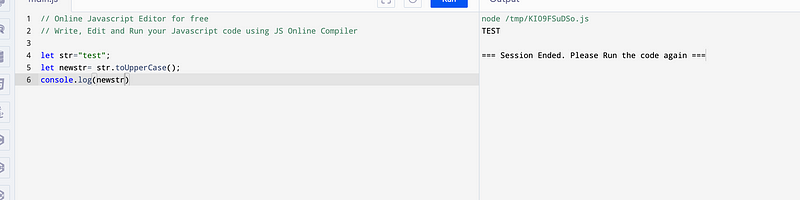
Section 1.2: Capitalizing the First Letter
Question 2: How can you capitalize the first letter of a string or sentence?
Understanding how to use the substring() method is key here.
Answer:
To capitalize the first letter, we can combine toUpperCase() with substring():
let str = "hello world"; // Only 'h' needs to be capitalized
let newStr = str[0].toUpperCase() + str.substring(1);
console.log(newStr); // Output: Hello world
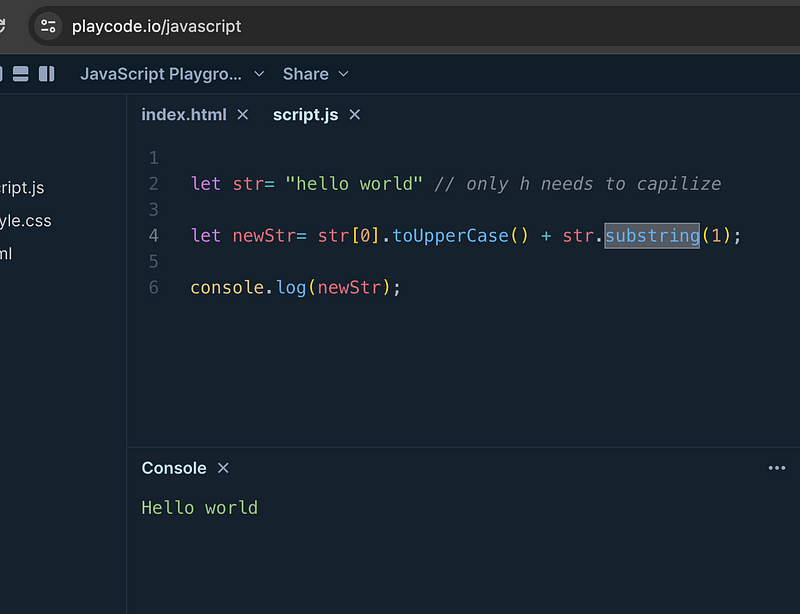
Section 1.3: Capitalizing Each Word
Question 3: How can you capitalize the first letter of each word in a sentence?
Answer:
We can utilize the split(), map(), and join() methods:
const str = "hello friends welcome to day 5";
const words = str.split(" ");
const capitalizedWords = words.map(word => word[0].toUpperCase() + word.substring(1)).join(' ');
console.log(capitalizedWords); // Output: Hello Friends Welcome To Day 5
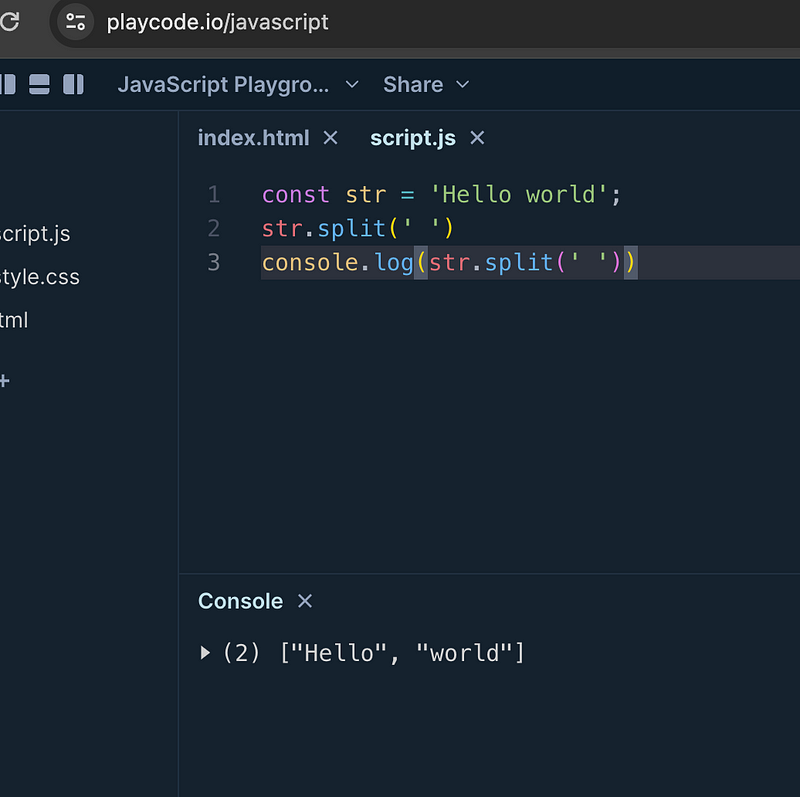
Now, you can also experiment with a for loop to achieve similar results!
Section 1.4: Reversing Strings
Question 4: How do you reverse a string?
A common interview question, reversing a string can be accomplished using the following approach:
Answer:
We can split the string into an array, reverse it, and then join it back together:
function reverseString(str) {
return str.split("").reverse().join("");
}
console.log(reverseString("hello")); // Output: olleh
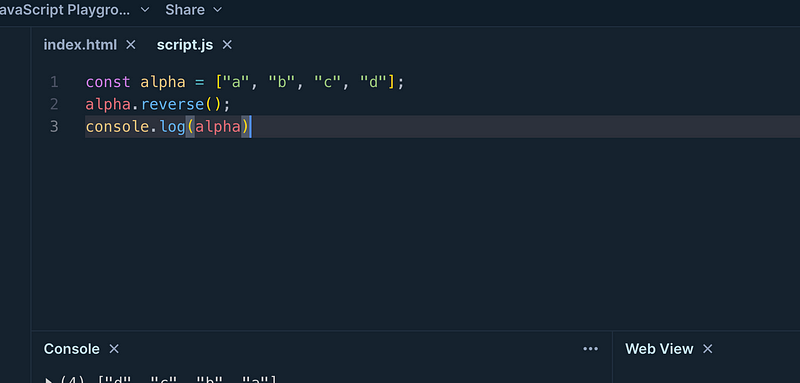
Section 1.5: Reversing Words
Question 5: How do you reverse the order of words in a string?
Answer:
To reverse the order of words, we can use:
function reverseWords(str) {
return str.split(' ').reverse().join(' ');
}
console.log(reverseWords('hello world')); // Output: world hello
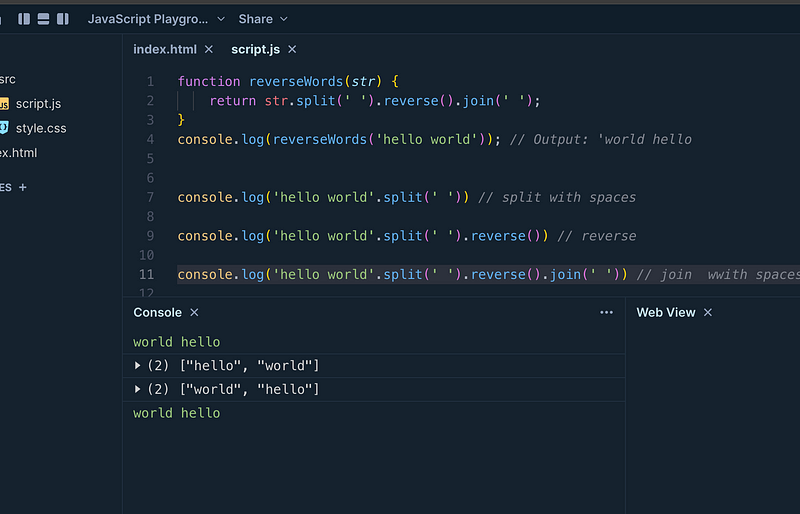
As we delve into string manipulation, remember that there's a vast landscape of possibilities. Continue exploring more examples over the next few days!
To further your understanding, check out these helpful videos:
The first video titled "5 JavaScript Concepts Every Beginner Developer Should Know" provides essential insights for those starting out.
The second video, "JavaScript Mastery Complete Course | JavaScript Tutorial For Beginner to Advanced," offers a comprehensive overview of JavaScript concepts.
Remember, sharing knowledge enriches us all. Stay kind and respectful in your learning journey!