Exploring Web Components with Angular Elements: A Comprehensive Guide
Written on
Chapter 1: Introduction to Web Components in Angular
In this guide, we will delve into the incorporation of Web Components within Angular, specifically utilizing Angular Elements. This offers a fantastic starting point for your projects!
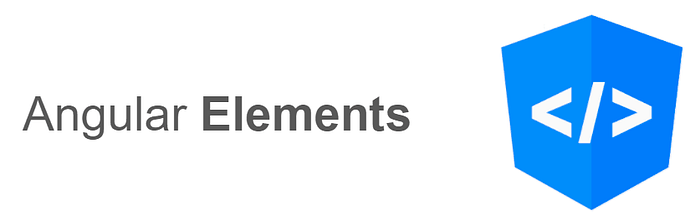
The focus of this article is on Angular Elements and Web Components. The realm of software development is filled with trendy terms, one of which is "micro front-end." But what does this concept entail? At its core, micro front-ends allow for the incremental release of various segments of your front-end application. Different teams can take charge of specific features, releasing them once they are ready. The introduction of Web Components has significantly facilitated this approach, enabling the creation of distinct components that can be seamlessly assembled to fit your application’s needs. Let's explore how this integration works in Angular. Throughout the article, you will encounter numerous code snippets, and a complete code repository will be accessible on GitHub, which I'll link to at the conclusion.
Section 1.1: Why Choose Angular?
Many readers may know of my enthusiasm for Angular. A key reason for selecting Angular Elements is its maturity as a Web Components library. It’s user-friendly and behaves similarly to any standard Angular project, with a few minor exceptions that we’ll discuss later. However, Angular isn’t the only option available. Web Components can be created using various technologies, including plain JavaScript, React, or Vue. For those who prefer alternatives to Angular, I recommend looking into StencilJS, developed by the Ionic team, which I plan to cover in a future article. StencilJS allows the use of plain JavaScript or any preferred library/framework, including Angular.
Section 1.2: Initiating the Project
One of the most challenging aspects of software engineering is naming your project. Once that hurdle is crossed, the next significant challenge is launching the project itself. Luckily, for our example project, these tasks are manageable. We will develop a simple Angular application comprising a single component: a contact form that collects a user’s name, email, and comments, complete with its own validation.
Starting a Web Components project in Angular follows the same procedure as any other Angular application. Since routing isn't necessary for this project, we can retain the default settings. Personally, I prefer to set the stylesheet processor to SCSS instead of CSS, but that choice is flexible. After creating your Angular project, open it in your preferred code editor, such as VS Code.
Next, we will incorporate Angular Elements.
This video titled "Using Web Components in an Angular project" provides a foundational overview and practical steps for using Web Components effectively within Angular.
Section 1.3: Configuring Angular Elements
Before diving into code, we need to perform some initial manual configurations. This section will guide you through setting up your application to generate a web component that can be exported anywhere.
Configuring Budgets
Initially, we need to adjust our budgets. But what exactly are budgets, and where do we locate them? When an Angular project is created, an angular.json file is generated, containing all the configurations necessary for building and serving the Angular application. This file includes a section labeled "budgets," which helps manage the size of your components and overall project build.
As your application grows, maintaining these budgets ensures that no single file becomes excessively large, which could hinder the build process. However, for our purposes, we want to configure it to output a single file instead of multiple smaller ones, so we will modify the budget settings.
We will remove the anyComponentStyle since we are essentially generating one large component, making it easier to manage styles. We will set the maximum warning limit to 2 megabytes and the maximum error limit to 5 megabytes for the initial budget.
You might be thinking that 5 megabytes seems quite high for a front-end project. While this is true, consider that:
- The maximum budget is unlikely to be reached; it merely serves as a warning and error threshold.
- The bundle will encapsulate all the functionalities that Angular provides without restrictions.
- Scripts can be deferred without noticeably impacting your application's performance.
Removing Output Hashing
We will also disable output hashing in the angular.json file. Output hashing is a useful Angular feature that appends a hash of file contents to the filename upon building. This allows the browser to cache files effectively, ensuring that users receive updates without delay. However, for Angular Elements, we need to turn this feature off for our components to function correctly.
Configuring the App Module
With our configurations set, it's time to modify the App Module. We will remove the bootstrap section under @NgModule to prevent the main component from bootstrapping like a typical application. We will also add CUSTOM_ELEMENTS_SCHEMA to the schemas array and configure the AppModule class accordingly.
Using Angular's Dependency Injection, we will create a custom element within the ngDoBootstrap function. The custom element will be designated as "contact-form." This simple example can be expanded to include multiple components if necessary.
Chapter 2: Building the Application
The video "Angular & Web Components - Using Angular Elements to create Custom Elements" dives deeper into how Angular Elements can be leveraged for creating custom web components.
Configuring the Build Step
At this point, if we were to execute the build command, multiple files would be generated in the dist folder. To ensure our web component functions correctly, we need all JavaScript files to be consolidated into one.
This can be accomplished by creating a concatenation script that runs during the build process. We will need to install a couple of development dependencies first. Afterward, we’ll create a file named concat.js in the root directory, which will consolidate runtime, main, and polyfill files into a single file called elements.js.
Testing Your Elements
Now that we have everything configured, testing is straightforward. We can comment out the <app-root> tag in the index.html file and replace it with our custom element. Once we start the application, we can confirm that everything is functioning correctly!
Creating the Contact Form
To create the contact form, we will utilize Angular Material. Although it’s not mandatory, it simplifies styling. After completing the setup, we can clear the app.component.html file and remove unnecessary variables.
Next, we will integrate Angular Flex-Layout to streamline layout management and import necessary modules in the app module. We will then establish a form group in the app component and create the HTML structure for the form.
With these elements in place, running the application should yield a fully functional form!
Exporting Data
To transmit form data to the website, we will utilize Angular Elements' capabilities. We will create an event emitter as an output and implement a submit function to relay form data upon submission.
Utilizing the Exported Component
Now that we have a working component ready for bundling, we can explore its real-world application. I recommend using http-server for easy local hosting. After setting up a test-website directory with an index.html file, we will import styles and scripts accordingly.
By appending an event listener to our custom component, we can capture data emitted from it, allowing for seamless integration into any site.
Final Thoughts
This overview has covered the integration of Web Components with Angular Elements. Although some complexities were omitted, the potential of Web Components is immense, especially in the context of micro front-end architecture.