Insights from My Two-Day Journey with React
Written on
Chapter 1: My Initial Encounter with React
In 2021, I took my first steps into the world of React. While my time with it was brief—just two days—I gathered some valuable insights that I’d like to share. It's important to note that I'm not an expert, and my experiences are simply personal observations.
Relax and enjoy the insights! Here are the five key points I found noteworthy.
Section 1.1: Understanding React
React is a powerful, declarative JavaScript library that excels at building user interfaces. It allows developers to create intricate UIs by combining smaller, isolated units of code known as "components," as explained by Slava Vaniukov, a leading expert and CEO of Softermii.
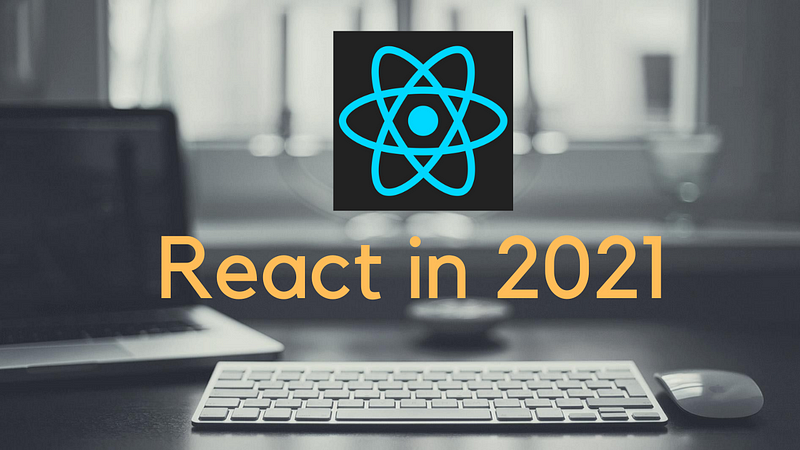
Section 1.2: Key Takeaways from My Experience
1. Minimal Learning Curve
I was pleasantly surprised by how straightforward it was to dive into React. Using the command line, I executed the following commands:
npx create-react-app my-app
cd my-app
npm start
After running these commands, I was greeted with a sample React application. I initially feared that the project structure would be overly complex, but to my relief, the essential configurations were minimal, allowing for easy adaptation as I progressed.
2. Instant Hot Reload
The hot reloading feature exceeded my expectations. After starting the local server, I made some minor modifications, such as changing the title, and the updates appeared almost instantly upon saving. The console even suggested installing extensions for React, which I found useful. However, I did discover that a server restart was necessary after adding new packages.
3. Component-based Architecture
When beginning to code a user interface, I instinctively broke it down into components, a practice that React actively promotes. I opted for functional components, which facilitated this approach.
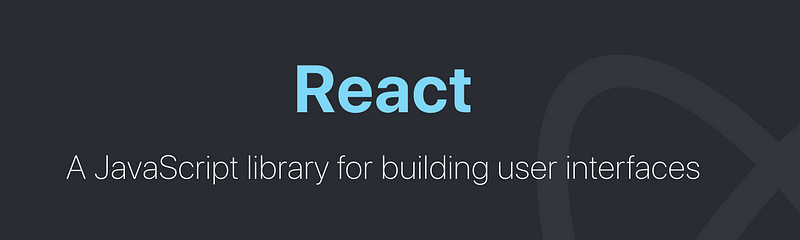
The crucial question is determining what should be a component. The principle of single responsibility comes into play here; each component should ideally perform a specific task. If a component begins to grow, it can be further divided into smaller subcomponents.
Example of a simple component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
Once established, components can be reused throughout the application.
4. Integration of JavaScript and Props
React's syntax closely resembles vanilla JavaScript, which likely contributes to its popularity. For instance:
const element = <h1>Hello, world!</h1>;
This isn’t HTML or traditional JavaScript; it’s JSX (JavaScript XML). When compiled, JSX translates into standard JavaScript function calls, resulting in JavaScript objects. Notably, React prefers camelCase property names, such as using className instead of class.
Props, or properties, enable data transfer between components, functioning similarly to HTML attributes. For example:
<Button onClick={btnOnClick} text="Fetch" />
Here, onClick and text are properties of the Button component. For type checking on these properties, React offers built-in capabilities through PropTypes, enhancing the robustness of the code.
Button.propTypes = {
text: PropTypes.string,
onClick: PropTypes.func.isRequired,
};
5. Enjoyable Development Experience
Ultimately, a positive development experience is crucial for the adoption of any technology. I found my time with React enjoyable and straightforward, which may explain its status as one of the leading frameworks today.
Other Notable Mentions
- Hooks (useState): A Hook is a special function that allows you to utilize React features. For instance, useState lets you manage state in function components.
import React, { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me</button>
</div>
);
}
Final Thoughts:
Every technology shares core concepts like loops and functions. The distinction lies in their syntax and structure, as well as how they facilitate logic implementation.
Chapter 2: Additional Resources
To further enhance your understanding of React, consider checking out the following resources.
The first video, "React Contributor Days | September 2021," provides insights from industry contributors about the React ecosystem.
The second video, "The BEST WAY to get started with React FOR BEGINNERS [2021]," is a fantastic introduction for newcomers looking to grasp the fundamentals of React.
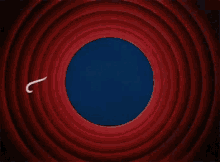