Mastering Form Validation in Vue 3 Using Vee-Validate 4
Written on
Chapter 1: Introduction to Form Validation
Form validation is a crucial aspect of application development. In this piece, we will explore the implementation of Vee-Validate 4 within a Vue 3 application for the purpose of validating forms.
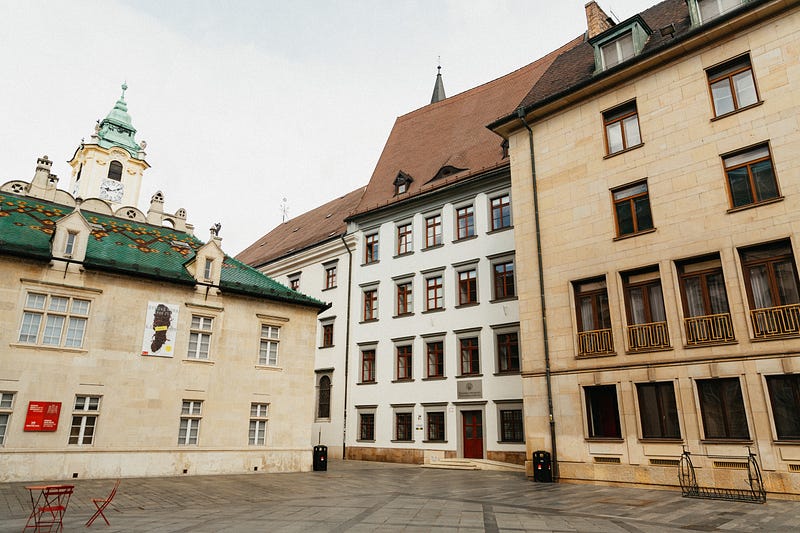
Section 1.1: Understanding the Minimum Length Rule
The 'min' rule ensures that the input value meets a specified minimum length. For example, the following code snippet demonstrates how to enforce a minimum character length of three:
<template>
<Form @submit="onSubmit" v-slot="{ errors }">
<Field name="field" rules="min:3" />
<span>{{ errors.field }}</span>
</Form>
</template>
<script>
import { Form, Field, defineRule } from "vee-validate";
import * as rules from "@vee-validate/rules";
Object.keys(rules).forEach((rule) => {
defineRule(rule, rules[rule]);
});
export default {
components: {
Form,
Field,
},
methods: {
onSubmit(values) {
alert(JSON.stringify(values, null, 2));},
},
};
</script>
We set a minimum value of 3, thus ensuring that the inputted data meets this requirement.
Section 1.2: Implementing Minimum Value Validation
Another useful feature is the min_value rule, which defines the smallest acceptable numeric value for input. Here’s how to use it:
<template>
<Form @submit="onSubmit" v-slot="{ errors }">
<Field name="field" rules="min_value:5" />
<span>{{ errors.field }}</span>
</Form>
</template>
<script>
import { Form, Field, defineRule } from "vee-validate";
import * as rules from "@vee-validate/rules";
Object.keys(rules).forEach((rule) => {
defineRule(rule, rules[rule]);
});
export default {
components: {
Form,
Field,
},
methods: {
onSubmit(values) {
alert(JSON.stringify(values, null, 2));},
},
};
</script>
If a user inputs a value below 5, they will receive an error notification.
Chapter 2: Numeric Input Validation
The numeric rule is essential for ensuring that the entered value is indeed a number. Here’s an example of how to implement this rule:
<template>
<Form @submit="onSubmit" v-slot="{ errors }">
<Field name="field" rules="numeric" />
<span>{{ errors.field }}</span>
</Form>
</template>
<script>
import { Form, Field, defineRule } from "vee-validate";
import * as rules from "@vee-validate/rules";
Object.keys(rules).forEach((rule) => {
defineRule(rule, rules[rule]);
});
export default {
components: {
Form,
Field,
},
methods: {
onSubmit(values) {
alert(JSON.stringify(values, null, 2));},
},
};
</script>
Additionally, you can use the following code to set validations dynamically:
<template>
<Form @submit="onSubmit" v-slot="{ errors }">
<Field name="field" :rules="validations" />
<span>{{ errors.field }}</span>
</Form>
</template>
<script>
import { Form, Field, defineRule } from "vee-validate";
import * as rules from "@vee-validate/rules";
Object.keys(rules).forEach((rule) => {
defineRule(rule, rules[rule]);
});
export default {
components: {
Form,
Field,
},
data() {
return {
validations: { numeric: true },};
},
methods: {
onSubmit(values) {
alert(JSON.stringify(values, null, 2));},
},
};
</script>
The first video, "Create a Login Form in Vue 3 with Vee-Validate 4," provides a comprehensive overview of creating a login form, emphasizing validation practices.
The second video, "Form Validation in Vue 3 using Vee-Validate," dives deeper into form validation techniques specific to Vue 3 applications.
Conclusion
In conclusion, Vee-Validate 4 offers robust tools for validating numeric input in your Vue 3 applications. For additional insights and examples, check out more resources at plainenglish.io.